Events and listening for events
<p>Events and listening for events</p>
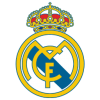
By Nabeel
Events and Listening for Events
Because phpVMS is using the Codon Framework, it has the ability to "throw" events. After you hook into the engine in your addon, you can "listen" for when an event is done, then do some actions based on which event.
To scan for an event, you create your add-on as usual. In your class constructor, you add a "listener", and then a function which is called everything an event is.
class MyModule extends CodonModule { // Register our module to listen for events public function __construct() { CodonEvent::addListener('MyModule'); } public function EventListener($eventinfo) { $eventinfo[0]; // Event name $eventinfo[1]; // Class calling it $eventinfo[n]; // Rest of the parameters (>= 2) } }
To view the list of events, view the [[events list]]
Adding the Hook
public function __construct() { CodonEvent::addListener('MyModule'); }
To add the hook, you use the function addListener():
CodonEvent::addListener(module_name, (optional) events);
- module_name - The name of your current module. This is needed to the engine knows where to direct
- events - optional array of the specific events to look for; it's only called if the events listed are called. Otherwise, it's called for every event:
// This will only register this module for the "login_success" event CodonEvent::addListener('MyModule', array('login_success'));
Catching the Event
After you've registered your module, you'll need to add the function to catch the event:
public function EventListener($eventinfo) { ... }
The $eventinfo parameter has the following:
- $eventinfo[**0**] - The event name
- $eventinfo[**1**] - Module that called it
- $eventinfo[**n**] - Additional parameters.
And example:
public function EventListener($eventinfo) { if($eventinfo[0] == 'login_success') { // Do something } elseif($eventinfo[0] == 'pirep_filed') { $pirepinfo = $eventinfo[2]; echo 'You filed a report from ' . $pirepinfo->depicao . ' to ' . $pirepinfo->arricao; } }
Stopping an Event
To stop an event, call the stop() function:
public function EventListener($eventinfo) { if($eventinfo[0] == 'pirep_prefile') { $pirepinfo = $eventinfo[2]; if($pirepinfo->aircraft == 'B737-800' && $pirepinfo->distance > 3000) { Template::Set('message', 'This aircraft distance is out of range for this flight!'); Template::Show('core_error.tpl'); CodonEvent::Stop(); } } }
In this example, it checks the PIREP to see if the aircraft is a 737-800 and the distance of the flight is invalid, meaning the aircraft is out of its operational range. We show the standard error message with the core_error.tpl (the standard error template), then we called CodonEvent::Stop(), which cancels the event from occuring.
The events which "obey" the stop command are noted, since not all events need it (in general, the events that occur before an action will obey a stop).
Events List
Here are a list of events which you can scan for. The parameters are listed as:
// Register our module to listen for events public function __construct() { CodonEvent::addListener('THIS_MODULE_NAME'); } public function EventListener($eventinfo) { $eventinfo[0]; // Event name $eventinfo[1]; // Class calling it $eventinfo[n]; // Rest of the parameters (>= 2) } // Example: public function EventListener($eventinfo) { if($eventinfo[0] == 'login_success') { // Do something } }
The Events
bid_preadd
Called before a bid has been added.
- $eventinfo[2] = ID of the schedule/route added
Codon::Stop() can be called during this event to stop the bid from being added.
bid_added
Called after a bid has been added.
- $eventinfo[2] = ID of the schedule/route added
login_success
This is called after a user logs in (when the login is successful).
pirep_prefile
This is called before a PIREP is filed.
- $eventinfo[2] = Values of the PIREP form
You can call CodonEvent::Stop() on this event to prevent the PIREP from being filed.
pirep_filed
This is called after a PIREP is filed (successfully)
- $eventinfo[2] = Values of the PIREP form
profile_viewed
This is called after the user views their profile.
Called from Profile module.
registration_precomplete
This is called before the registration is added.
- $eventinfo[2] = The user's registration data
The stop function (CodonEvent::Stop()) can be called to cancel the registration.
registration_complete
This is called after a successful registration is completed.
Called from the Registration module
- $eventinfo[2] = The user's registration data
Recommended Comments
There are no comments to display.
Join the conversation
You can post now and register later. If you have an account, sign in now to post with your account.