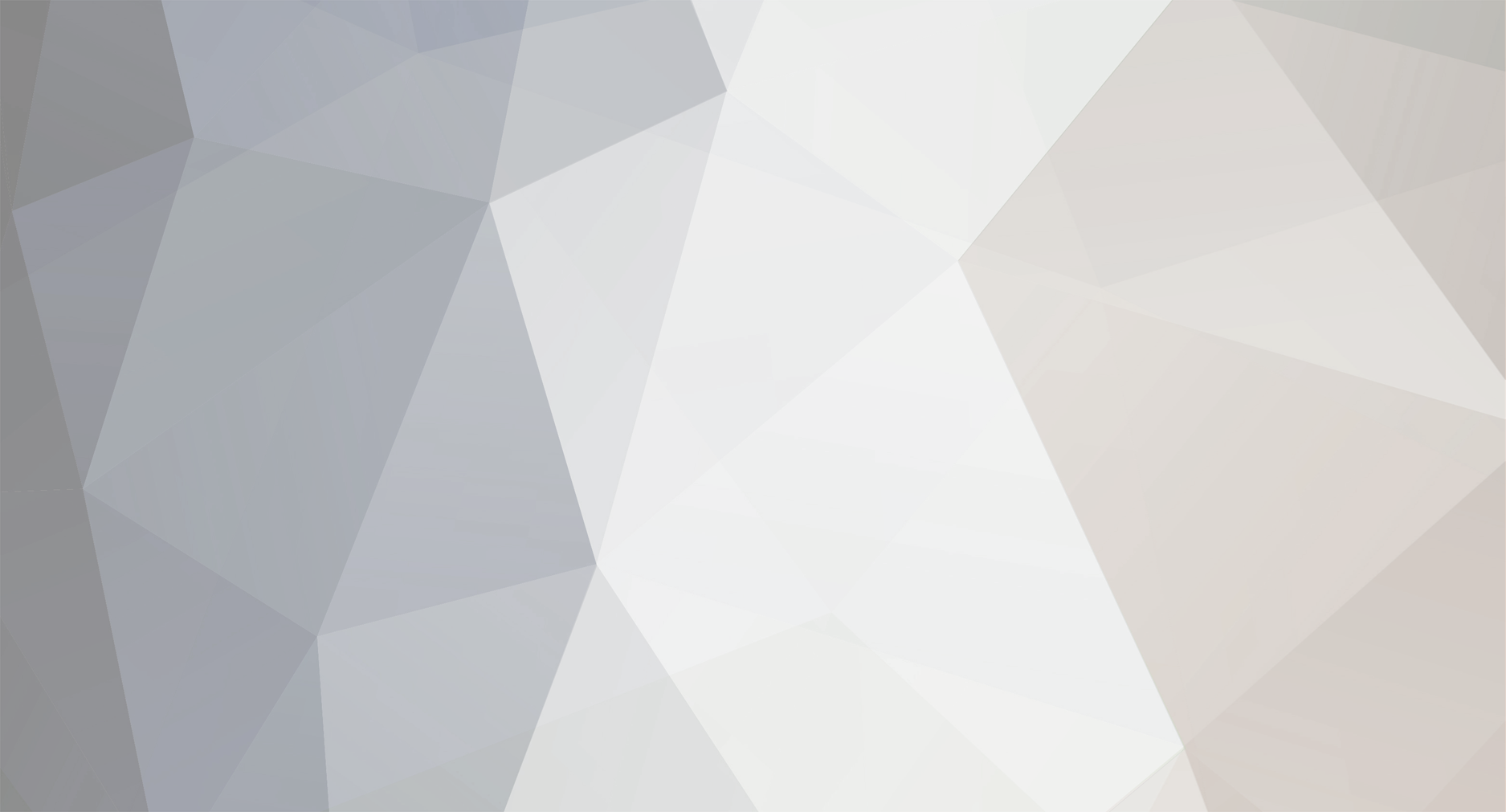
web541
Members-
Posts
700 -
Joined
-
Last visited
-
Days Won
17
Content Type
Profiles
Forums
phpVMS Resources
Downloads
Everything posted by web541
-
Was this on the search page or after they have jumpseated? If it's on the search form page, get the pilot to log out and log back in again (or refresh the page). If it's after they have jumpseated then make sure you've uploaded all the files correctly and uploaded the 'fltbook.sql' file to your database correctly.
-
If it's just a static page then yes it's possible (if it's dynamic, as in it requires a lot of database activity/a lot of methods then it's best put through a controller/module) For this, I'll just create a static about page 1. Go to resources/views/layouts/default (unless you have another layout, then put it in there) 2. Create a new folder called 'about' 3. Create a new file inside this folder called 'index.blade.php' 4. Inside this file place this @extends('app') @section('title', 'About') @section('content') <!-- Content HTML goes here --> <h3>Test</h3> @endsection Notes: The @extends('app') means that this page will be extending off the master template called 'app.blade.php' which is found in the resources/views/layouts/default folder The @section('title', 'About') means that (because it is set by the @yield('title') in the master template) this will show up in the <title></title> of the browser tab. In this case we've set it to 'About'. The @section('content') is where you put your normal HTML for your page (this is also set in the master template) 5. Routing Go to app/Routes/web.php and find this line (around line 17) Route::get('livemap', 'AcarsController@index')->name('livemap.index'); The ::get means you are submitting a GET HTTP request to the url '/livemap' (note this can be inside a prefix) The 'AcarsController@index' means you are targeting the 'index' method (function) of the AcarsController controller The ->name('livemap.index'); is giving this route a unique name (in this case 'livemap.index') and can be referred to in the app via route('livemap.index') After this line, place this Route::get('about', function() { return view('layouts.default.about.index'); })->name('about_page'); Where the view file being targeted is located in resources/views/layouts/default/about/index.blade.php (hence the layouts.default.about.index) The principles are the same, but it's using a 'Closure' (the function() bit) instead of passing it through a controller, because it is just a static page and there are no other methods being used, this is sufficient. 6. Go to {http://yourvaurl.com}/about and it should show up. Final notes: I suggest you read the docs over at http://docs.phpvms.net/customizing and also the laravel blade docs over at https://laravel.com/docs/master/blade to get used to the new templating system. When updating the phpVMS version you may lose this work, so for that reason I recommend making a module or widget. Most of the principles are the same in terms of the actual templating but the routing won't affect the default files (instead it is put inside the module). Check out the docs here http://docs.phpvms.net/developers/add-ons-and-modules for info about that. If there are multiple pages you want added, you could make a module that contains all of them (either by storing the HTML in the database or by routing the templates through a controller) which would be easier than making a module for each page.
-
<?php $aircraft = OperationsData::getAircraftInfo($schedules->aircraftid); echo $aircraft->icao; ?> Change $schedules->aircraftid to the aircraft id of your table, provided the id's match between the aircraft table and your table then it should work.
-
Is this what you're looking for? <?php echo OperationsData::getAircraftInfo($schedules->aircraftid); ?> <?php echo OperationsData::getAircraftByName($schedules->aircraft); ?>
-
Try this <?php $pilots = DB::get_results("SELECT * FROM ".TABLE_PREFIX."pilots ORDER BY `totalpay` DESC"); $ranking = 0; foreach($pilots as $p) { echo '#'.$ranking.' | '.PilotData::getPilotCode($p->code, $p->pilotid).' - $'.$p->totalpay; echo '<br />'; $ranking++; } ?>
-
I wouldn't consider it more up to date than 5.5.x (it was Nabeel's v2 ported up which was quite old to begin with) but still usable. The intention was to make the current version work with PHP 7.0 so I would assume that it would run fine on that setup (as 5.5.x has never been given the official 'run on PHP 7.0+'). As for security, it's possible that some minor adjustments (for PHP 7.0) here and there have been added but nothing too major should have been impacted (otherwise we would have been notified). The modules depend on the developer, I'd say most would work fine but you might have a few hiccups if they have some deprecated features or otherwise. If you already have a working site then I'd personally wait for v7 than to try and mess around with upgrading again.
-
Oh right I know what the issue is now. In your first post, I took it as the aircraft itself wasn't showing up for each schedule (I have made a setting for that in the admin panel) as it was blocked off, but really it was the schedule that was blocked off instead so therefore the aircraft were also blocked off. Yes the DISABLE_SCHED_ON_BID should be set to false, if it is set to true then the app takes it and means "Yes I want to disable the bid if someone else has got one" but if it is set to false then it means "No I don't mind if someone else bids on the same schedule". If you want a clean install, then check here.
-
This is quite odd, if you change the value to 'No', does it update (it could be that the settings table isn't read properly) and does it allow the aircraft to be booked by multiple people? I just tested the module on a fresh install and it worked first time for me. In the event that it still doesn't work, you can go into core/templates/fltbook/confirm_bid.php and delete lines 27-31 which should force it to unlock if something is wrong.
-
If you go to the admin panel and to the settings for this module, what is this set to? If you change it, does it work? Allow aircraft to be booked if someone else has booked a schedule with that aircraft
-
The SQL error is because you already have a column name called 'airline' in your 'phpvms_aircraft' table. This could be from another custom module, or from you importing the SQL file more than once. Can you go to phpmyadmin => structure of the the 'phpvms_aircraft' table and screenshot it. Also take one of your aircraft table (only the first row will be fine). Also, in your core/local.config.php file, what is this set to? DISABLE_SCHED_ON_BID
-
phpVMS Version 2 (https://github.com/nabeelio/phpvms_v2) was the original phpVMS version and still works to some extent, but requires your PHP version to be >5.6. phpVMS Version 5.5.x (https://github.com/DavidJClark/phpvms_5.5.x) is the current stable version of phpVMS and can be used on PHP systems (mainly on 5.5.12) of up to 5.6. Some people have made it work on PHP v7 with no errors, but it's different for everyone on their own hosting. phpVMS Version 7 (https://github.com/nabeelio/phpvms) is currently in development and is a totally different version of the two listed above. This version will be the newest and most up-to-date version when released but is still somewhere between Alpha and Beta stages (you can test it though, but there will still be bugs and changes that will occur before the official release). This version is made for PHP Versions >7.1.
-
1. Try this <?php $pendingpireps = count(PIREPData::findPIREPS(array('p.accepted' => PIREP_PENDING))); $pendingpilots = count(PilotData::findPilots(array('p.confirmed' => PILOT_PENDING))); echo ($pendingpireps + $pendingpilots); 2. smartCARS doesn't report the rawdata on its own. You could modify their web scripts and try and make it work, but this depends if the developer has hardcoded anything on the actual app's side or not.
-
<?php //VAForum Original by:simpilot //VAForum Security updates + VAForum 2.0 onwards by: Tom Sterritt ?> <?php $this->show('forum_nav.tpl'); ?> <h3>New Post</h3> <script src="/lib/js/ckeditor/ckeditor.js"></script> <div style="text-align: left;"> <form action="<?php echo url('/Forum');?>" method="post" enctype="multipart/form-data"> <table width="80%" align="left"> <tr> <td>Posted By:</td> <td align="left"><b><?php echo Auth::$userinfo->firstname.' '.Auth::$userinfo->lastname?></b></td> </tr> <tr> <td>RE: </td> <td align="left"><?php $topic = ForumData::get_topic_name($topic_id); $name = $topic->topic_title; echo $name; ?></td> </tr> <tr> <td>New Post</td> <td align="left"><textarea class="ckeditor" rows="10" cols="50" name="message" value=""></textarea></td> </tr> <tr> <td colspan="2"> <input type="hidden" name="topic_id" value="<?php echo $topic_id; ?>" /> <input type="hidden" name="forum_id" value="<?php echo $forum_id; ?>" /> <input type="hidden" name="pilot_id" value="<?php echo Auth::$userinfo->pilotid; ?>" /> <input type="hidden" name="action" value="submit_new_post" /> <input type="submit" value="Submit Post"> </td> </tr> </table> </form> </div> Give that a try.
-
Try this <table width="100%"> <?php echo '<td width="250px" valign="top">'; echo '<table cellspacing="1" cellpadding="1" border="1">'; echo '<th width="150px"><div align="left">Country Location</div></th>'; echo '<th width="100px"><div align="center">Pilots</div></th>'; $country_info = DB::get_results('SELECT COUNT(pilotid) as total, location FROM '.TABLE_PREFIX.'pilots GROUP BY location LIMIT 10'); foreach($country_info as $country) { echo '<tr>'; echo '<td align= "left">'; echo '<img src="'.Countries::getCountryImage($country->location).'" /> '; echo Countries::getCountryName($country->location); echo '</td>'; echo '<td align="center">'; echo ' ('.$country->total.')'; echo '</td>'; echo '</tr>'; } echo '</table>'; ?> </table>
-
Has this always been happening, or is it just with the new skin? Also, can you pm me the link to your site.
-
VAForum 2 Deprecated: Non-static method ForumData etc...
web541 replied to in2tech's topic in Support Forum
Go into core/common/ForumData and find all of these public function and replace them with this public static function and that should fix it. -
Check that your browser console doesn't have any errors (could be a jQuery issue). Also, check that the forms have an method of POST not GET <form action="xxxx" method="post"> ... </form>
-
The local.config.php doesn't need to be changed too much, but rather a few other files. Have you followed the tutorial here? https://www.virtualairlines.eu/index.php/Blog
-
Hmmm, never seen this one before. In lib/fonts, do you have a file called Silkscreen.ttf? That's where the local.config.php points to for this under Config::Get('SIGNATURE_FONT_PATH'); Fonts directory should look like this https://github.com/DavidJClark/phpvms_5.5.x/tree/master/lib/fonts Also, check that the permissions on the file and the fonts folder are set correctly.
-
Have you checked your browser console? Could be a jQuery issue as Bootstrap 4 uses a higher version than phpVMS does.
-
Are you sure that .BoundsBounds and .Bounds are functions in the js Google Maps API? They might be but last time I checked they weren't. And also, there is no need to use an iframe in this circumstance due to the nature of how phpVMS works. Are you running PHP 7.0 at the moment?
-
The 'phpvms' API server is currently down (or at least responds differently to phpvms < 7.0), you can either use this or just wait until phpVMS v7.0 is released (I believe you can fiddle with it in alpha at the moment) Another option is to manually add each airport from an online source and it should work as normal if you don't have too many to add.
-
Inside core/common/AutoAssignData, find all of these public function change them to this public static function And that should work. If it doesn't, then it's being called elsewhere and you'll have to wait for a reply considering the module is payware.
-
Ah ok that's much easier then. Have you skinned before? Try this link The template you're using might be slightly different in the way things are displayed and linked, but as long as the phpVMS variables are in there, it should work as normal. If you have tried to skin it, what problems have you had?